CS 174: Introduction To Object Oriented And Systems Programming
Ursinus College, Spring 2022
Instructor: Christopher J. Tralie
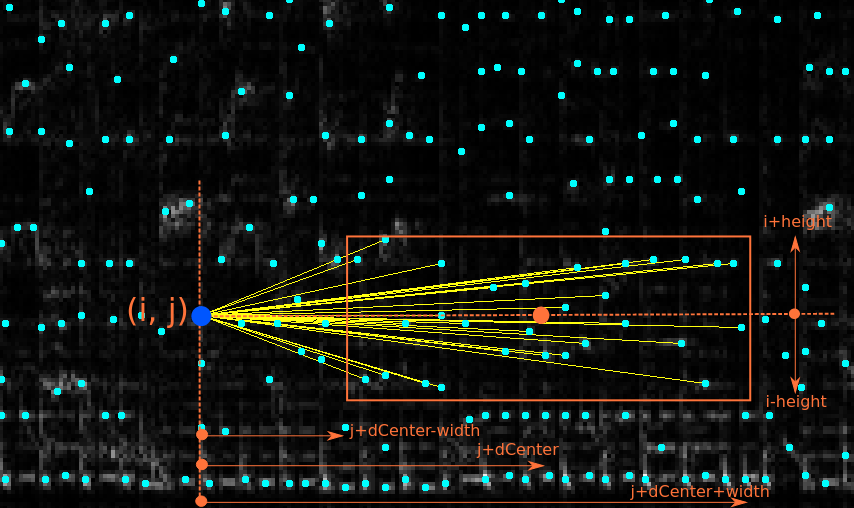
A conceptual drawing of the Shazam algorithm, which is used to identify music from small clips of digital audio. Students will use object oriented concepts to implement this as their final project in the class.
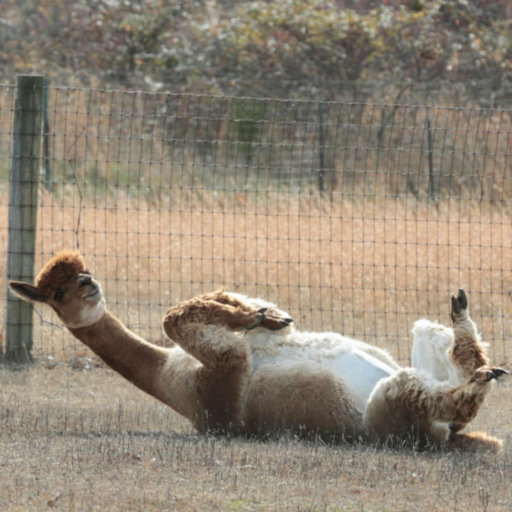
3 short low quality digital music clips are hidden in this image (original photo taken by my cousin Allie Mellen). Students will learn how to extract this kind of hidden data using concepts from systems programming.
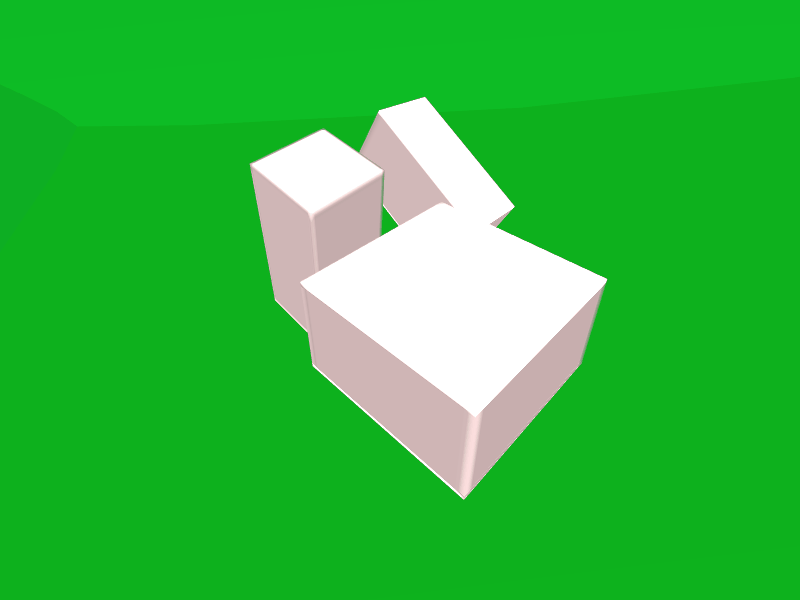
Tralie World, by Michael Kates, CS 174 Fall 2020
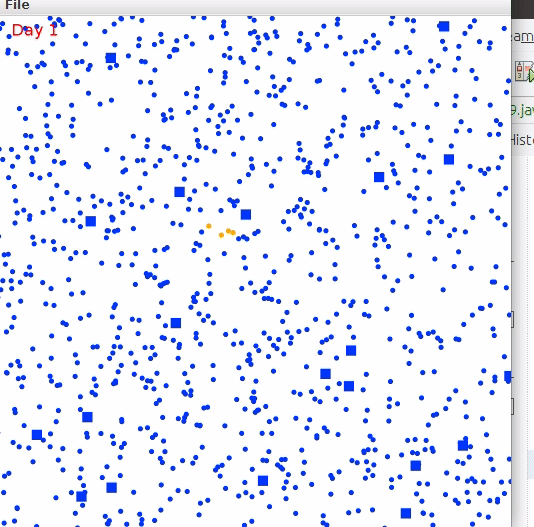
A simple Monte Carlo simulation of the spread of a pandemic throughout a population, which students will implement in this course.
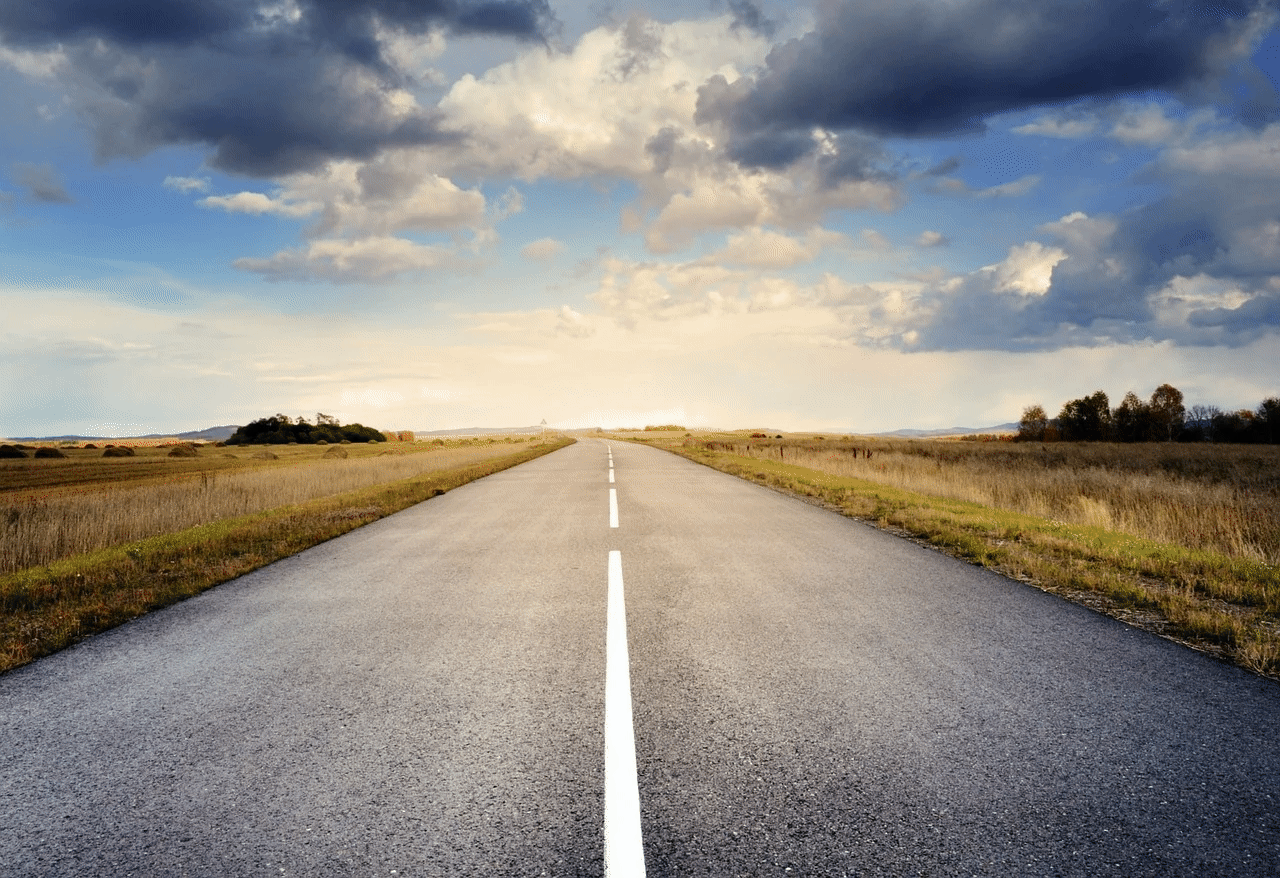
An algorithm that performs Canny edge detection and the Hough transform to detect where the road and the horizon likely are in an image (this could be used, for instance, in autonomous driving). These are examples of embarrassingly parallelizable algorithms, which students will learn to accelerate using threads in C++.
Table Of Contents
Overview
Class Times / Locations
- Monday and Friday, 11AM - 12:15 PM, Wednesday 11AM - 11:50AM in Pfahler 109
Student Office Hours
-
Monday/Wednesday 3:30PM - 4:30PM in Pfahler 210
-
Wednesday/Friday 10AM - 11AM in Pfahler 215
-
Tuesday 12PM - 1PM virtually on Discord
Prerequisites/Requirements
CS 173 with a grade of C- or better, or permission of the instructor.
Instructor
Christopher J. Tralie
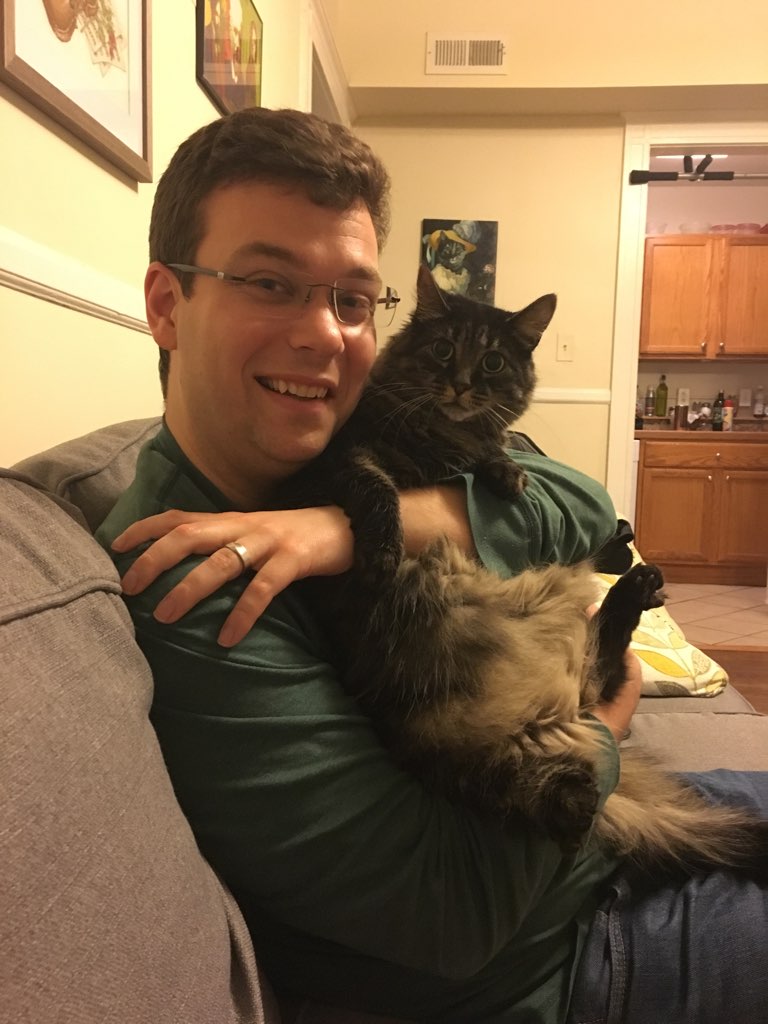
I grew up right around the corner in the Montgomery County and attended Upper Dublin High school (class of 2007). I then did my undergraduate degree in Electrical Engineering at Princeton University and my master's and Ph.D. degrees in Electrical And Computer Engineering at Duke University (heavily studying math and CS along the way). I finally started my dream job at Ursinus College in Fall of 2019! You can read more about my interests on my professional web site. Looking forward to getting to know everyone as we work through this course together!
Office Hours / Helproom T.A.
Tom Boccuto
Monday/Wednesday 4:30PM - 5:30PM, And Occasionally on Discord
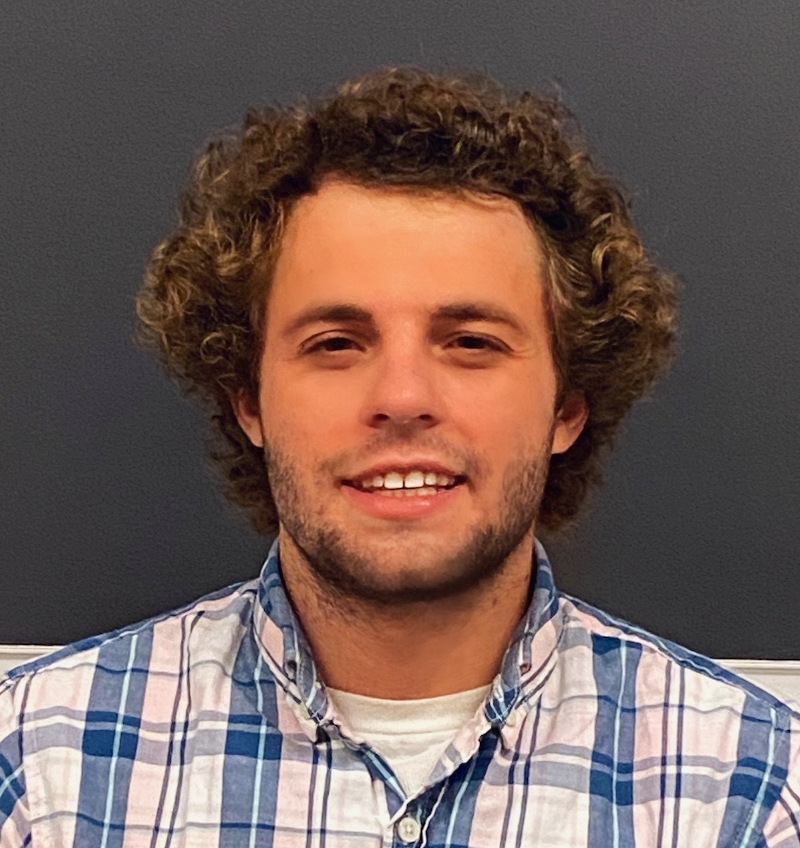
Tom is a 174 alumnus and a serious C++ enthusiast. He'll be around to provide additional help with the labs and assignments. I've intentionally overlapped him with my Monday/Wednesday office hours so we will both be around at points, and so that he can continue to help people after I leave those days.
Course Description
This course is the continuation of CS 173, and it can be seen as the second part of an introductory computer science and introductory programming sequence.
Now that students are familiar with the fundamentals of programming, such as variable types, conditional statements, loops, and methods, the class will branch out into two more sophisticated directions: systems programming and object oriented programming. This class will prepare students for more advanced work in computer architecture and data structures, respectively.
In systems programming, we look "under the hood" at how programs are really run on the computer, digging into things such as memory (de)allocation, or where variables actually get stored, and metaprogramming, or the process of automating and running code from the command line. For this reason, our language of choice in this class will be C++, which has syntax very similar to Java, but which requires a lot more care managing memory and program compilation.
In object oriented programming, on the other hand, we zoom out focus more on the design of programs at a high level. The goal is to make programs that are easy to read and maintain by others. At the core of object oriented programming are classes, or their instances: objects (hence, the name). While we always have primitive variable types, such as int
, double
, and char
at our disposal, a class can be though of as a "designer variable type." For example, we can make a "Person" class that stores the name, age, and height of a person. That might look something like this in C++:
An instance of a class is known as an object. Just like 10
is an example of an int
, chris
is an example of a person, whose name is "Chris", whose age is 31, and whose height is 6.08 feet. In this way, we have encapsulated (i.e. "placed in a capsule") what it means to be a person all in once place. This makes it way easier to define multiple people, as we don't have to define separate variables for their name, age, and height each time. Moreover, we can start to create instance methods in a class that can be used to change the state of a particular object. For instance, a method void doBirthday()
could increment the age of a person by 1 (e.g. chris.doBirthday()
would change the age of the chris
object to 33).
Objects allow us to enforce two principles that make software much more usable and easier to maintain: modularity and reusability. Modularity refers to the ability to encapsulate everything doing related tasks under one umbrella, while reusability refers to versatile, general purpose code that doesn't have to be completely rewritten for minor changes. Both of these properties should be apparent from any well-executed object oriented design.
As was emphasized in CS 173, a high level programming language like C++ gives us an intuitive, organized way to talk to computers, but computer science is automation and computing in general, not tied to a specific language. In this course, we will be exploring C++, but the focus is on programming in general in the service of a broad, interdisciplinary array of applications. A few examples of programs students will be working on include
- Creating virtual models of 3D cities.
- Steganography, or the process of hiding data in one format in plain sight in another format.
- Creating a COVID-19 simulation which includes quarantined areas and medics.
- Implementing parts of the "Shazam algorithm" to automatically determine what song someone is listening to based on a small clip of audio.
Learning Goals
- Implement and engineer multi-component systems that solve real world problems.
- Write software that is well-organized with good encapsulation and which is easy to read and maintain by others.
- Begin to develop a sense for algorithm efficiency and the synergy between algorithms and data structures.
- Demistify what's going on "under the hood" when code runs on your computer.
Learning Objectives
- Learn the basics of "metaprogramming," or writing scripts to compile code, in the command line.
- Practice patient problem solving by developing comfort with the edit -> compile -> run loop, along with intermediate debugging skills.
- Make appropriate design choices between compile time (e.g. static members of a class and compile time memory) and runtime code structures (e.g. object member variables and dynamic memory).
- Allocate and clean up dynamic memory, avoiding memory leaks.
- Implement data structures, such as queues, linked lists, trees, and hash tables, using object-oriented paradigms.
Technology Logistics
We will be using a zoo of technologies in the course, as has become standard in 21st century work environments. Below is a table summarizing what kinds of communications/activities occur via each technology, and below that there are more details on everything. This is admittedly complex, and it will take some getting used to, but it will be worth it once we get it nailed down.
NOTE: I will repeat the same announcements across e-mail and Discord, so you don't have to check all both for announcements.
Class web site (You are here!) |
|
Canvas |
|
Discord |
|
Microsoft Teams |
|
|
*: For privacy reasons, anything of a personal nature, and particularly things that have to with educational records (e.g. grades), need to be kept within Ursinus sanctioned platforms like Outlook e-mail and Microsoft Teams.
Canvas
We will be using Canvas, but only to submit assignments and to store all of the grades. I will also keep all of the due dates current on the calendar there, as students have appreciated this common space for all of their classes in the past.
Discord
To facilitate informal, class-wide discussions about the class, as well as buddy group coding with screen sharing, we will have a Discord channel for the class. My goal is for this to turn into a flourishing area to work through confusion and to share ideas as a group. All questions are welcome! To help break down the barrier of asking questions, we will be using the chat bot Voltaire so students can ask questions anonymously. This has worked very well in the past. We will also keep the CS 174 Discord channel open during class for polling and for general questions for students who are shy about speaking up verbally in front of their peers.
In addition to the regular channel, we will be holding drop-in student office hours on Tuesdays on the Ursinus ACM Discord channel, since student office hours are joint between my classes. You can find it under the "Chit Chat" Category, as shown below
Do not send me direct messages or anything of a sensitive nature (e.g. grades) over Discord. Instead, use Microsoft Teams or e-mail for that, since those transactions are locked down better under Ursinus control.
Microsoft Teams
For one on one direct messages with me, we will be using Microsoft Teams, which is linked to your Office suite through Ursinus, so you are automatically enrolled.
Readings
There is no official textbook for this course. Instead, I will sometimes link to online resources on the schedule, or I will have you read something or watch a video and complete exercises before class for initial exposure to some topics. I will also write and share notes on the schedule to summarize things that we did in class, as needed.
Deliverables
Programming Assignments
The bulk of the grade in the course will be earned by completing 5 medium scale programming assignments and one large scale one (the "Shazam algorithm" at the end). Be sure to start them early, since debug time can often be unpredictable! Refer to the collaboration and sharing rules for these assignments.
Labs
As in CS 173, we will have a number of labs throughout the course. Generally, our 30 minute Wednesday blocks will be devoted to these, and final submissions will be due the following Monday. Collaboration is more open here, and I will generally provide more starter code relative to the programming assignments, since the idea is for students to get some initial exposure and to practice ideas before moving onto more complicated things.
Debugging Principles
If you're taking this course, then you've certainly had experience with debugging, but it is a skill you will still need to work on, so you should expect to hit some roadblocks. In fact, it is time consuming and difficult even for very experienced programmers. So do not be hard on yourself if your programs don't work the first time around (they rarely do, even if you've been programming for decades!).
I have had nearly 20 years of programming experience at this point, and I have learned the hard way what works and what doesn't. Here are my main debugging principles in a nutshell
- Leave yourself adequate time to work on the assignments, because the amount of time it takes to resolve issues can be unpredictable.
- Write small bits of code and test them right away. Don't write a wall of code and test it, only to find out that something doesn't work. By contrast, if you write bits at a time, you will know right away what code you wrote caused things to be wrong.
- Apply the scientific method: have in mind hypothesis for what might be wrong, design a quick experiment to test your hypothesis, draw conclusions, and repeat.
- Fail quickly. If you're working on a larger scale program that processes a lot of data, do not wait for several minutes for data to load every time you make a small change. Instead, come up with the minimum, simplest experiment you possibly can which will tell you whether your code is correct or not.
- Don't forget that you can write code to help you automate debugging. Otherwise, it's sometimes tedious to repeat the same steps over and over again as you're changing things.
- Know when to walk away. We often get stuck in loops wanting to resolve things, but then our logical thinking goes out the window and we start randomly trying different things. Even if you're up against the clock, it is often good to take a little break and come back again a little bit later.
Class Engagement / Pre-Class Work
It's easier to learn difficult subjects like object oriented and systems programming by going through a number of examples on each topic beyond the examples in the large assignments. Because of this, we will often do small programming exercises and experiments in class related to a topic that we're learning. At times, I may ask you to read over a description of an exercise or to read some notes about a new topic before class so we can go more deeply into the examples. I may also ask you to hand in something small related to the class exercises to make sure you were putting effort in during class. This will all make up a small part of your grade in the class.
Schedule
Outlined below is the schedule for the course, including lecture topics and assignment due dates. All assignments are due at 11:59PM on the date specified. The specific dates of different topics are subject to change based on the pace at which we go through the course.
Lecture | Lectures (click for notes) | Readings/Links | Assignments/Deliverables | |
Unit 1: Introduction To C/C++ And Systems Programming | ||||
1 | Wed (50 mins) 1/19/2022 | Course Overview, C Primitives and printf, Loops, Conditionals, Arrays | ||
Thu 1/20/2022 | Survey/Syllabus Quiz Due | |||
2 | Fri (75 mins) 1/21/2022 | The Terminal, Filesystem Navigation | C++ Fundamentals Module Due before Class | |
3 | Mon (75 mins) 1/24/2022 | C++ Practice (10 Heads in A Row), Header files | Assignment 0: Software Test Due
Assignment 1 (Individual): Design Your Own City Out | |
4 | Wed (50 mins) 1/26/2022 | Makefiles, Lab 1: HTML Holidays | ||
5 | Fri (75 mins) 1/28/2022 | Pointers | Pointers Fundamentals Exercises Due Before Class | |
6 | Mon (75 mins) 1/31/2022 | C Pointers And Arrays, Dynamic Memory |
| Lab 1 Due |
7 | Wed (50 mins) 2/2/2022 | Lab 2: C++ Bug Hunt, LLDB |
| |
8 | Fri (75 mins) 2/4/2022 | 2D Arrays, 2048 Game | Assignment 1 Due | |
9 | Mon (75 mins) 2/7/2022 | Dynamic Memory, Valgrind | Binary And Hex Module Due Before Class | |
Tue 2/8/2022 | Lab 2 Due | |||
10 | Wed (50 mins) 2/9/2022 | Command Line Arguments, 2D Array Allocation/Cleanup | Assignment 2 (Buddy): Image Processing And Steganography Out | |
11 | Fri (75 mins) 2/11/2022 | Binary, Hex, Bitwise Operators | ||
Unit 2: Fundamentals of Object Oriented Programming | ||||
12 | Mon (75 mins) 2/14/2022 | Introduction To Classes: Constructors, Instance Variables / Methods | ||
Tue 2/15/2022 | Assignment 2 Check-In | |||
13 | Wed (50 mins) 2/16/2022 | C++ Classes practice and header files | ||
14 | Fri (75 mins) 2/18/2022 | C++ Classes: Static Variables, Information Hiding/Encapsulation (public and private) | ||
15 | Mon (75 mins) 2/21/2022 | Lab 3: Drawing Shapes with Aggregation And Inheritance | CS 174: Classes And Inheritance Modue Due Before Class | |
Tue 2/22/2022 | Assignment 2 Due | |||
16 | Wed (50 mins) 2/23/2022 | Begin Polymorphism | ||
Thu 2/24/2022 | Assignment 3 (Buddy): Invasive Species Polymorphism Out | |||
17 | Fri (75 mins) 2/25/2022 | Finish Polymorphism, STL Linked Lists | Lab 3 Due | |
18 | Mon (75 mins) 2/28/2022 | Abstract Classes Lab 4: Comparators And Insertion Sort | ||
19 | Wed (50 mins) 3/2/2022 | Multiple Inheritance, The Diamond Problem | ||
20 | Fri (75 mins) 3/4/2022 | Catch-Up Day | Assignment 3 Part 1 Due
Lab 4 Due | |
Unit 3: Object-Oriented Data Structures | ||||
-- | Mon (75 mins) 3/7/2022 | Spring Break | No CS 174 Class. Enjoy the break! | |
-- | Wed (50 mins) 3/9/2022 | Spring Break | No CS 174 Class. Enjoy the break! | |
-- | Fri (75 mins) 3/11/2022 | Spring Break | No CS 174 Class. Enjoy the break! | |
21 | Mon (75 mins) 3/14/2022 | Linked List Implementations with Nodes/Aggregation | ||
Tue 3/15/2022 | Linked List Module Due | |||
22 | Wed (50 mins) 3/16/2022 | Lab 5: My Vector (Dynamic Array ADT) | ||
23 | Fri (75 mins) 3/18/2022 | Continue Linked Lists | ||
24 | Mon (75 mins) 3/21/2022 | Linked Lists / Stacks | Assignment 3 Due
Assignment 4 Part 1(Buddy): Doubly Linked Lists Out | |
Tue 3/22/2022 | Lab 5 Due | |||
25 | Wed (50 mins) 3/23/2022 | Doubly-Linked Lists, Queues | Last Day To Drop with A "W" | |
26 | Fri (75 mins) 3/25/2022 | STL Priority Queue, Prim's Algorithm | ||
27 | Mon (75 mins) 3/28/2022 | STL Set, STL Map, Begin LinkedMap Implementation | ||
28 | Wed (50 mins) 3/30/2022 | LinkedMap Implementation | Assignment 4 Part 1 Due | |
Thu 3/31/2022 | Linked Map Module Due | |||
29 | Fri (75 mins) 4/1/2022 | Hash Tables | Assignment 5 (Buddy): Basketball Hashing Out | |
30 | Mon (75 mins) 4/4/2022 | Recursion And Memoization: Factorial, Fibonacci, Ackermann | ||
31 | Wed (50 mins) 4/6/2022 | Lab 6: Recursive Drawing / Fractals | Ackermann Exercise 1 Due | |
32 | Fri (75 mins) 4/8/2022 | Binary Search Trees: Cleanup, Addition, Traversal with Preorder/Inorder/Postorder | Ackermann Exercise 2 Due | |
33 | Mon (75 mins) 4/11/2022 | Binary Search Trees: Drawing | Lab 6 Due | |
34 | Wed (50 mins) 4/13/2022 | Lab 7: Huffman Trees | ||
35 | Fri (75 mins) 4/15/2022 | Continue Huffman Trees, Merge Sort | Assignment 5 Due | |
Unit 4: Advanced Topics in Systems Programming | ||||
36 | Mon (75 mins) 4/18/2022 | Function Pointers | ||
Tue 4/19/2022 | Lab 7 Due | |||
37 | Wed (50 mins) 4/20/2022 | Intermezzo: Digital Audio And Shazam | Final Assignment (Individual): Shazam Out | |
38 | Fri (75 mins) 4/22/2022 | Shazam Continued | ||
39 | Mon (75 mins) 4/25/2022 | Begin Parallel Programming And Threads | ||
40 | Wed (50 mins) 4/27/2022 | Race Conditions And Mutexes | ||
41 | Fri (75 mins) 4/29/2022 | Lab 8: Image Filtering with Threads | ||
42 | Mon (75 mins) 5/2/2022 | Work Session / Classes In Java | ||
Fri 5/13/2022 | Final Assignment: Shazam Due |
Grading
Breakdown
Class Engagement / Pre-Class Work | 15% |
Labs | 25% |
Programming Assignments | 60% |
Flexible Submission Policy
The purpose of deadlines is to keep students on track as they work through the course to avoid a snowball effect, so students should communicate with me early if they don't think they will make the posted deadlines (even if, and especially if, they have accommodations), and we can discuss a plan together. In the absence of said communications, all assignments are due at 11:59PM EST on the date(s) stated on the schedule. Students can turn in those assignments past the deadlines, and the scores will be adjusted as follows:
- -5% for work submitted between 1 minute - 6 hours late
- -10% for work submitted up to 12 hours late
- -15% for work submitted up to 24 hours late
- -25% for work submitted up to 48 hours late
- -40% for work submitted up to 96 hours late
- -50% for work submitted more than 96 hours late
Letter Grades
Letter grades will be assigned on the scale below at the end of the course.
|
|
|
|
|
Classroom Environment
Inclusive Environment
Computer science is a field that has historically been and continues to be steeped in inequalities. We will do our best to put the topics we're working on into the appropriate historical context, and to address broader societal issues that are related to the code that we write. We will strive to do better in our course, with an honest look at where we have been in the field. To that end, my goal is to foster a environment in which students across all axes of diversity feel welcome and valued, both by me and by their peers. Axes of diversity include, but are not limited to, age, background, beliefs, race, ethnicity, gender/gender identity/gender expression (feel free to tell me in person or over e-mail which pronouns I should use), national origin, religious affiliation, and sexual orientation. Discrimination of any form will not be tolerated.
.Furthermore, I want all students to feel comfortable expressing their opinions or confusion at any point in the course, as long as they do so respectfully. As I will stress over and over, being confused is an important part of the process of learning computer science. Learning computer science and struggling to grow is not always comfortable, but I want it to feel safe. In other words, I will regularly keep you at the boundary of your comfort zone with challenging, real-world assignments, but I want you to feel comfortable with me and your peers and respected as a learner during the process.
Finally, I am aware that, particularly during the pandemic, there are a variety of factors that may make it difficult to perform at your best level in class. At Ursinus, we are fortunate to have quite a mix of students from different backgrounds, many of whom need to work part time, and an increasing number of whom are commuters and have family obligations. If you find yourself having difficulty performing at the level that you want and/or turning assignments in on time because of any of these issues, communicate with me, and we can come up with a solution together (I will gently reach out if I notice any slips even if you don't communicate). This is a foundational course for the CS major, and I want to work to keep your excitement alive, regardless of your personal circumstances. You belong in CS!
Participation
Classroom Attendance And Etiquette
Students are expected to attend class in person. We're shooting for engagement over mere attendance; students are expected to be active in class exercises and to be fully invested in the class (i.e. no internet browsing). Students who are unable to attend class for significant reasons (whether isolation or quarantine for students who have received a positive test, those experiencing Covid-related symptoms while awaiting test results, or other issues that make it difficult to attend class) should work pro-actively to make up any class exercises that they missed. To help with this, I will do my best to put up Youtube videos from myself and other instructors, as well as class notes, on topics that we cover.
Finally, students are expected to follow any college policy requiring mask wearing on campus, in addition to following any guidance faculty provide for their individual classes. Masks should be available in every academic building, if needed.
Maximizing Your Communal Experience
Here are ways students can maximize their experience as a class community, and which could lead to extra credit in certain situations.
- Helping to teach a student a topic during office hours.
- Certain calls for participation in class
- Particularly helpful or insightful messages on Discord
- Finding mistakes in the book or on the assigned homework
Discord Communication Policy
Since this is a class-wide communication, the following rules apply to online communication- Students are expected to be respectful and mindful of the classroom environment and inclusivity standards. They are equally applicable to a virtual environment as they are in class.
- Students are not permitted to publicly share direct answers or questions which might completely give away answers to any homework problems. When in doubt, send me a direct message on Microsoft Teams.
- I will attempt to answer questions real time during student office hours. Otherwise, I will make every attempt to respond within 24 hours on weekdays. I cannot be expected to respond at all on Saturdays or Sundays or outside of 10AM-8PM on weekdays, so plan accordingly. (Of course, students can and should still respond to each other outside of these intervals, when appropriate).
The points above are part of a more general term referred to as "netiquette." Refer to the chart below, provided by Touro College
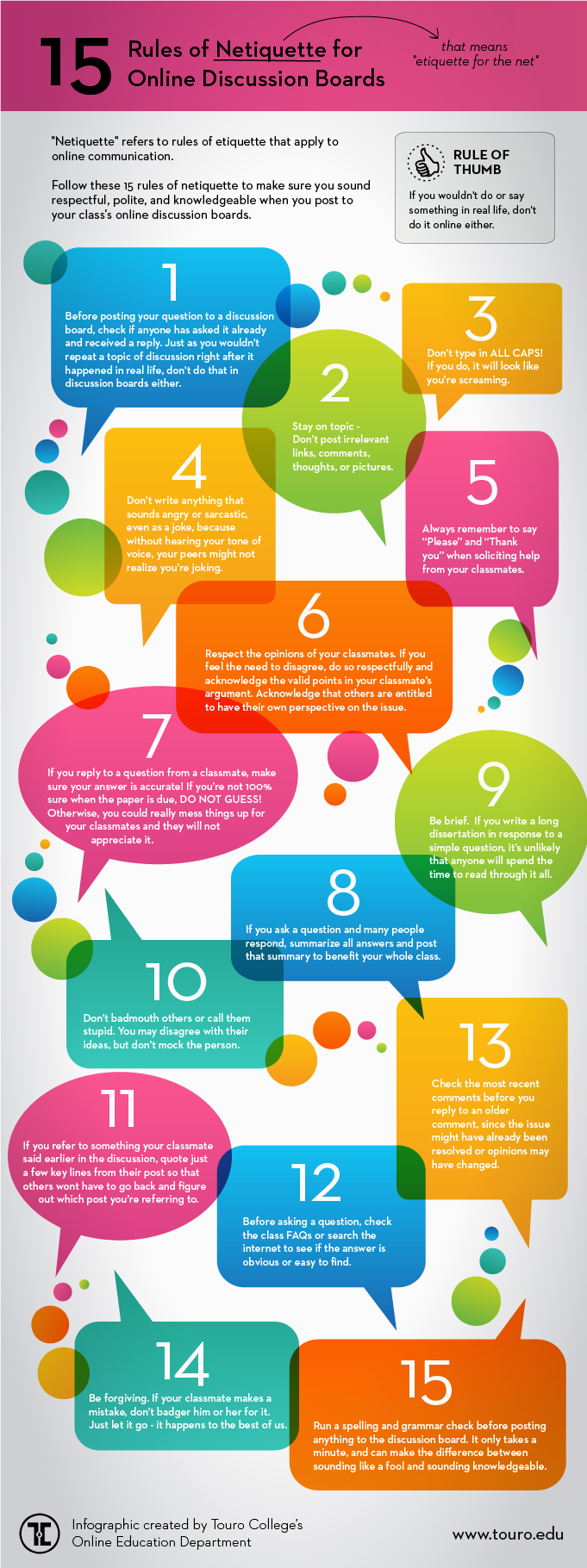
Collaboration Policy
Overall Philosophy
The collaboration policy for this class walks the line between encouraging openness and collaboration during a challenging learning process, while also making sure that each students is progressing technically at an individual level without relying on 100% on other classmates. Communication between students is allowed (and encouraged!) on most assignments, but it is expected that every student's code or writeups will be completely distinct. Do not copy code off of the Internet. Do cite any sources in addition to materials linked from the course website that you used to help in crafting your code and completing the assignment.
Assignment Buddies
To encourage collaboration, students will be allowed (not required) to choose one or more "buddies" to work "near" during assignments and labs. Students are still expected to submit their own solutions, but they are allowed to provide substantial help to each other, and even to look at each others' code during the process. Students should indicate their buddies in the README upon assignment submission. Let me know if you would like a buddy but are having trouble finding one.
For the labs, collaboration rules are slightly more permissive beyond buddies. See the collaboration grid below.
Individual Assignments
There will be a few assignments that students are expected to complete on their own with no communication with anyone but me. They will be limited in number, but this will be just to make sure every individual students is progressing technically. You can think of them like an open-ended, open-book take-home quiz where you can ask me questions.
Collaboration Scenarios Table
Below is a table spelling out in more detail when and how you are allowed to share code with people (table style cribbed from Princeton CS 126).
Click on each button below to view the collaboration parameters for each scenario. Labs are more permissive than assignments, which are more permissive than individual tasks
Lab Collaboration Grid
YOUR BUDDY |
COURSE STAFF |
CS 174 GRADS |
CLASS- MATES |
OTHER PEOPLE |
|
---|---|---|---|---|---|
DISCUSS CONCEPTS WITH: | ✔ | ✔ | ✔ | ✔ | ✔ |
ACKNOWLEDGE COLLABORATION WITH: | ✔ | ✔ | ✔ | ✔ | ✔ |
EXPOSE YOUR CODE/SOLUTIONS TO: | ✔ | ✔ | ✔ | ✔ | ✘ |
VIEW THE CODE/SOLUTIONS OF: | ✔ | * | ✘ | ✔ | ✘ |
COPY CODE/SOLUTIONS FROM: | ✘ | * | ✘ | ✘ | ✘ |
Assignment Collaboration Grid
YOUR BUDDY |
COURSE STAFF |
CS 174 GRADS |
CLASS- MATES |
OTHER PEOPLE |
|
---|---|---|---|---|---|
DISCUSS CONCEPTS WITH: | ✔ | ✔ | ✔ | ✔ | ✔ |
ACKNOWLEDGE COLLABORATION WITH: | ✔ | ✔ | ✔ | ✔ | ✔ |
EXPOSE YOUR CODE/SOLUTIONS TO: | ✔ | ✔ | ✔ | ✘ | ✘ |
VIEW THE CODE/SOLUTIONS OF: | ✔ | * | ✘ | ✘ | ✘ |
COPY CODE/SOLUTIONS FROM: | ✘ | * | ✘ | ✘ | ✘ |
Individual Collaboration Grid
YOUR BUDDY |
COURSE STAFF |
CS 174 GRADS |
CLASS- MATES |
OTHER PEOPLE |
|
---|---|---|---|---|---|
DISCUSS CONCEPTS WITH: | N/A | ✔ | ✘ | ✘ | ✘ |
ACKNOWLEDGE COLLABORATION WITH: | N/A | ✔ | ✘ | ✘ | ✘ |
EXPOSE YOUR CODE/SOLUTIONS TO: | N/A | ✔ | ✘ | ✘ | ✘ |
VIEW THE CODE/SOLUTIONS OF: | N/A | * | ✘ | ✘ | ✘ |
COPY CODE/SOLUTIONS FROM: | N/A | * | ✘ | ✘ | ✘ |
* You may view and copy code from class exercises and class resources without citing them, but you should not copy solutions from previous semesters that the instructor may have provided
NOTE: The terms "exposing" and "viewing" exclude sending or ingesting electronically, which would be considered copying. Exposing and viewing are normally done in the context of in-person working or in the help room. Since we are working remotely, what this means is that buddies can screen share as they are working through things, but they should not send code directly.
NOTE ALSO: "Other people" includes internet sources.
If the collaboration policy has been violated in any way, regardless of intent, then it may be an academic dishonesty case, and it will be referred to the Associate Dean for Academic Affairs. I am required to make this report in every occurrence, so it is best to speak with me first if there are any questions about the policy or expectations. You should feel free to have these conversations with me anytime prior to making your submission without fear of penalty.
On a more personal note, though a willful violation of academic honesty may seem merely transactional to a student, faculty take violations very personally, as they are disrespectful to the time and effort we put into our courses. I would also like to emphasize that your reputation is much more important than your grades. The recommendations we as faculty write go a long way, and we are much happier to write positive recommendations for students with lower grades who show grit and growth than we are to write recommendations for students with higher grades who have cheated.
Other Resources / Policies
Accommodations
In addition to our general awareness diversity, Ursinus College is also committed to providing reasonable accommodations to students with disabilities. Students with a disability should contact the Directory of Disability Services ASAP. Dolly Singley is located in the Center for Academic Support in the lower level of Myrin Library. Visit this link for more information on the process. I will do my best to accommodate your requests, and they will be kept completely confidential.
One on One Tutoring
One on one tutoring for up to two hours per week is available through the institute for student success. Please click here to fill out a Qualtrics survey if you'd like to take advantage of this.
Let's Talk
Mental health care is increasingly recognized as a crucial service for the undergraduate population. Please visit this link for more information about complementary counseling services provided by the college. The Wellness Center has a virtual drop-in crisis hour at 2-3 pm each weekday, which is available for students in crisis who need to be seen immediately by a clinician. If you are still hesitant to go, take me (Professor Tralie) as an example of someone who has benefited greatly from talk therapy in the past. I am happy to discuss this in office hours in more detail.
Beyond that, please have a look at this document for a variety of resources related to mental health at Ursinus.
Finally, be aware that there are resources outside of mental health care proper to address some core sources of mental stress and strain, such as time management and writing at the Institute for Student Success and the Center for Writing And Speaking.
Title IX
Title IX is a federal law, under which it is prohibited to discriminate on the basis of gender. The Title IX Coordinator is available to receive inquiries and to investigate allegations in this regard.
Inclement Weather Policy
In the event that the College closes due to inclement weather or other circumstances, our in-person class sessions, drop-in office hours, or other meetings will not be held. I will contact you regarding our plan with regard to rescheduling the class or the material, any assignments that are outstanding, and how we can move forward with the material (for example, any readings or remote discussions that we can apply). If necessary, I may schedule online virtual sessions in lieu of class sessions, and will contact you with information about how to participate in those. I will communicate this plan to the department so that it can be posted on my office door if it is feasible to do so. This policy and procedure will also apply in the event that the College remains open but travel conditions are hazardous or not otherwise conducive to holding class as normal. Should another exigent circumstance arise (for example, illness), I will follow this policy and procedure as well.